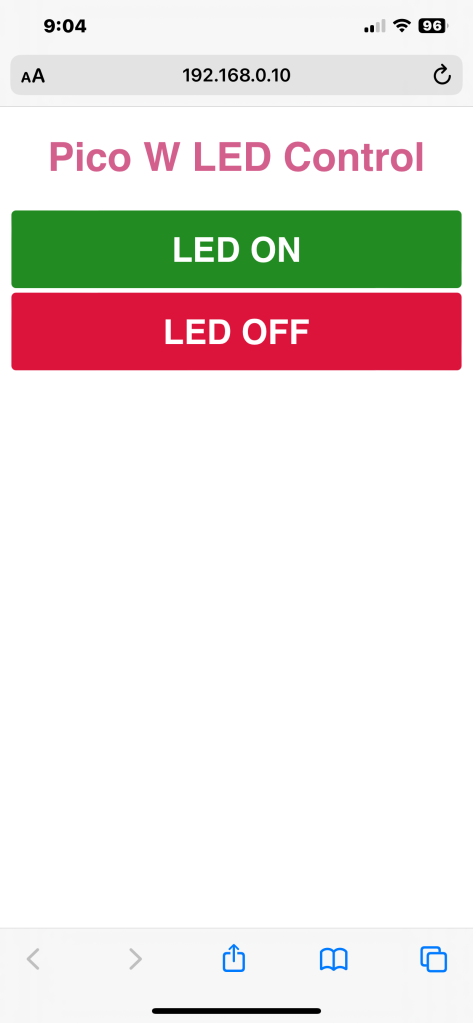
Monday 18 March, Adafruit released version 9 of CircuitPython. I had been working with version 9 pre-releases up to release candidate 1, but due to some problems with RC1 I dropped back to 8.2.10. I might try version 9 on another different board I have, but for now I’m sticking with 8.2.10 until such time as I actually have to migrate to version 9 in the future.
The code I’m presenting came from an Adafruit project over a year ago, and I can’t recall where it was, but it was complicated with additional device control. I’ve taken the code and tidied it up a bit, especially the embedded HTML that’s used to render the webpage on a smartphone’s web browser. I’ve tested it on both Android as well as iOS, and it works fine regardless.
Note that I’ve also used the new CircuitPython’s feature of settings.toml to define not just the local SSID and password, but the static IP address of the CircuitPython webserver as well as if it’s running in debug mode or not. It’s a lot easier to change a small toml file than to go and try to change something in the code body.
Once up and running all you need to do is either turn on the on-board LED or turn it off. If you have a serial application attached to the USB port, then you’ll see debug information streaming out the port. This is because DEBUG on line 26 has been set to True in the settings.toml file.
And one more thing: in debug mode, you navigate to [STATIC_IP_ADDRESS]:5000 to see the page. When not in debug mode, then the webserver is on regular port 80.
import osimport timeimport ipaddressimport wifiimport socketpoolimport boardimport microcontrollerfrom digitalio import DigitalInOut, Directionfrom adafruit_httpserver import Server, Request, Response, POST# On-device LED setupled = DigitalInOut(board.LED)led.direction = Direction.OUTPUTled.value = Falseipv4 = ipaddress.IPv4Address(os.getenv('STATIC_IP'))netmask = ipaddress.IPv4Address("255.255.255.0")gateway = ipaddress.IPv4Address(os.getenv('GATEWAY'))wifi.radio.set_ipv4_address(ipv4=ipv4,netmask=netmask,gateway=gateway)wifi.radio.connect(os.getenv('AP_SSID'), os.getenv('AP_PASSWORD'))print(" Connected to WiFi access point...")pool = socketpool.SocketPool(wifi.radio)server = Server(pool, "/static", debug=os.getenv('DEBUG'))font_family = "sans-serif"# The HTML page body setup as an 'f' string.## Use {{ and }} if something within HTML *actually* needs to be in brackets# such as CSS style formatting.#def webpage():html = f"""<!DOCTYPE html><html><head><meta http-equiv="Content-type" content="text/html;charset=utf-8"><meta name="viewport" content="width=device-width, initial-scale=1"><style>html{{font-family: {font_family};background-color: #FFFFFF;display:inline-block;margin: 0px auto;text-align: center;}}h1{{color:#D35F8D;word-wrap: break-word;font-size: 35px;}}p{{font-size: 1.5rem;word-wrap: break-word;}}.button-red{{font-family: {font_family};display: inline-block;width: 99%;background-color: #DC143C;border: none;border-radius: 4px;color: white;padding: 16px 40px;text-decoration: none;font-size: 30px;margin: 2px;cursor: pointer;}}.button-green{{font-family: {font_family};display: inline-block;width: 99%;background-color: #228B22;border: none;border-radius: 4px;color: white;padding: 16px 40px;text-decoration: none;font-size: 30px;margin: 2px;cursor: pointer;}}p.dotted {{margin: auto;width: 90%;font-size:25px;text-align: center;}}</style></head><body><title>Pico W LED Control</title><h1>Pico W LED Control</h1><form accept-charset="utf-8" method="POST"><button class="button-green" name="LED ON" value="ON" type="submit">LED ON</button></a><button class="button-red" name="LED OFF" value="OFF" type="submit">LED OFF</button></a></form></body></html>"""return html# route default static IP@server.route("/")def base(request: Request): # pylint: disable=unused-argumentreturn Response(request, f"{webpage()}", content_type='text/html')# Determine which button was pressed and execute associated action.#@server.route("/", POST)def buttonpress(request: Request):raw_text = request.raw_request.decode("utf8")print(raw_text)## If the led on button was pressed...if "ON" in raw_text:led.value = True## If the led off button was pressed...if "OFF" in raw_text:led.value = Falsereturn Response(request, f"{webpage()}", content_type='text/html')print(" Starting HTTP server...")try:server.start(str(wifi.radio.ipv4_address))print(f" Listening on http://{wifi.radio.ipv4_address}")## If the server fails to start then kick-start the Pico W.#except OSError:time.sleep(5)print(" !!! OSERROR RESTARTING !!!")microcontroller.reset()print(" Start polling...")is_healthy = True;## DO NOT hard code as True, instead provide an exit if something goes wrong.#while is_healthy:try:# Poll for incoming requests.server.poll()# pylint: disable=broad-exceptexcept Exception as e:print(e)is_healthy = Falsecontinue
AP_SSID = "YOUR_SSID"AP_PASSWORD = "YOUR_SSID_PASSWORD"STATIC_IP = "192.168.0.10"GATEWAY = "192.168.0.1"DEBUG = "True"
You must be logged in to post a comment.