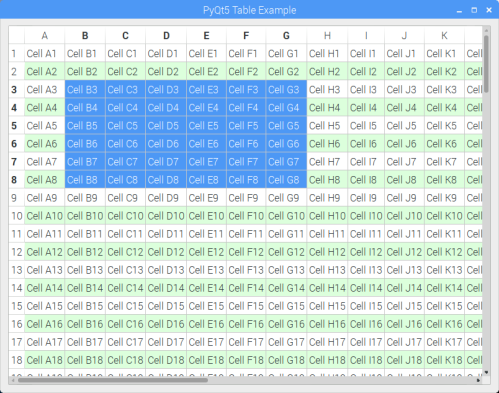
I’m still learning about PyQt5 on the Raspberry Pi. In this little experiment I’m learning how to create a simple table using QTableWidget, similar to a spreadsheet. This example shows
- How to invoke it
- How to connect to various event triggers
- How to manage the look of the table
The code is a bit longer than my other posts so far, weighing in at 113 lines. And it’s noticeably incomplete. But it’s still work publishing at this point, if for no other reason that if I don’t do it now I don’t know when I will, because I don’t know if or when I’ll finish it enough to be useful. But as an example, it’s useful enough for those who might need an idea or two.
What I’ll note so far is that the events triggered while manipulating the cells in the table are a bit peculiar. The only event that fires first, and reliably, is the cellPressed event. It will fire every time the cell is selected with a left mouse button down. Right after you get the cellClicked() event, most of the time. But I’ve seen instances where cellClicked() isn’t fired 100% of the time.
The cell contents are all modifiable, and if you make a change and hit return, then the cellChanged() event is reliably fired every time. My next steps are to add menus and right-mouse-button enabling for editing capabilities. And I’m trying to determine how to programatically determine the range of multiple cell selection as the screen cap shows at the beginning. What comes out is very peculiar and not reliable. I’m beginning to wonder if there isn’t a bug in the PyQt5 QTableWidget.
#!/usr/bin/env python3import sys, osfrom PyQt5.QtWidgets import (QApplication,QWidget,QTableWidget,QTableWidgetItem,QVBoxLayout)from PyQt5.QtGui import QColorclass App(QWidget):def __init__(self):super().__init__()self.setWindowTitle('PyQt5 Table Example')self.setGeometry(100, 100, 800, 600)# Create a table, create a box layout, add the table to box layout and# then set the overall widget layout to the box layout.#self.createTable()self.layout = QVBoxLayout()self.layout.addWidget(self.tableWidget) self.setLayout(self.layout) self.show()# Create a table with alphabet header labels. We're attempting to mimic# the classic spreadsheet look. This goes back to VisiCalc on the Apple ][# introduced in 1979.#def createTable(self):## Define the max number of rows and the colomns. Lable the columns# with letters of the alphabet like spreadsheets since VisiCalc.#self.maxRows = 99self.headerLabels = ["A","B","C","D","E","F","G","H","I","J","K","L","M","N","O","P","Q","R","S","T","U","V","W","X","Y","Z"]self.tableWidget = QTableWidget()self.tableWidget.setRowCount(self.maxRows)self.tableWidget.setColumnCount(len(self.headerLabels))self.tableWidget.setHorizontalHeaderLabels(self.headerLabels)# Pre-populate the cells in the spreadsheets with data, strings in# this example.#for row in range(0, self.maxRows):for col in range(0, len(self.headerLabels)):self.tableWidget.setItem( row, col,QTableWidgetItem("Cell {0}{1}".format(self.headerLabels[col], row+1)))## Set every other row a light green color to help readability.#if row % 2 != 0:self.tableWidget.item(row,col).setBackground(QColor(220,255,220))self.tableWidget.move(0,0)## The next two function calls 'tighten up' the space around the text# items inserted into each cell.#self.tableWidget.resizeColumnsToContents()self.tableWidget.resizeRowsToContents()# Hook various events to their respective callbacks.#self.tableWidget.cellClicked.connect(self.cellClicked)self.tableWidget.cellChanged.connect(self.cellChanged)self.tableWidget.cellActivated.connect(self.cellActivated)self.tableWidget.cellEntered.connect(self.cellEntered)self.tableWidget.cellPressed.connect(self.cellPressed)# This is executed when the user clicks in a cell.#def cellClicked(self):for currentQTableWidgetItem in self.tableWidget.selectedItems():print(' Clicked:', currentQTableWidgetItem.row(), currentQTableWidgetItem.column(), currentQTableWidgetItem.text())def cellChanged(self):for currentQTableWidgetItem in self.tableWidget.selectedItems():print(' Changed:', currentQTableWidgetItem.row(), currentQTableWidgetItem.column(), currentQTableWidgetItem.text())def cellActivated(self):for currentQTableWidgetItem in self.tableWidget.selectedItems():print(' Activated:', currentQTableWidgetItem.row(), currentQTableWidgetItem.column(), currentQTableWidgetItem.text())def cellEntered(self):for currentQTableWidgetItem in self.tableWidget.selectedItems():print(' Entered:', currentQTableWidgetItem.row(), currentQTableWidgetItem.column(), currentQTableWidgetItem.text()) def cellPressed(self):for currentQTableWidgetItem in self.tableWidget.selectedItems():print('Pressed:', currentQTableWidgetItem.row(), currentQTableWidgetItem.column(), currentQTableWidgetItem.text())if __name__ == '__main__':app = QApplication(sys.argv)ex = App()print('PID',os.getpid())sys.exit(app.exec_())
You must be logged in to post a comment.